Status: Complete
Type: Game Modification, Automation
Technology: Lua, Minecraft, Computer Craft
This is a case of simulated automation using video games as a tool to make scenarios to support making your own versions of technology that might otherwise be unfeasible. In this case I’m using Minecraft to support the automation of machinery/mechanical processes. Using a mod called Computer Craft I can have agents in the world called Turtles (which maybe a reference to some classic ways of teaching certain programming concepts). Turtles can do a lot of things that players can do, such as manipulate inventories, mine items, craft items, etc. making them very useful if you’re able to understand and support their abilities.
In this case I have two different programs designed for them. One was originally supposed to take input from a chest and divide charcoal between two other chests while redirecting anything else to another chest for further processing. This was defunct pretty quickly since I later implemented my second program to alleviate the need for the first.
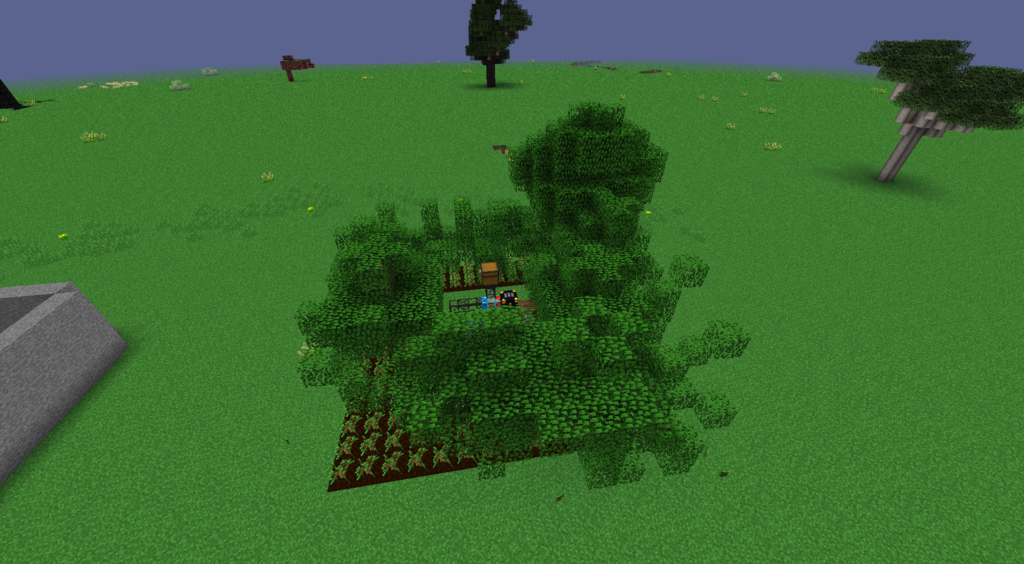
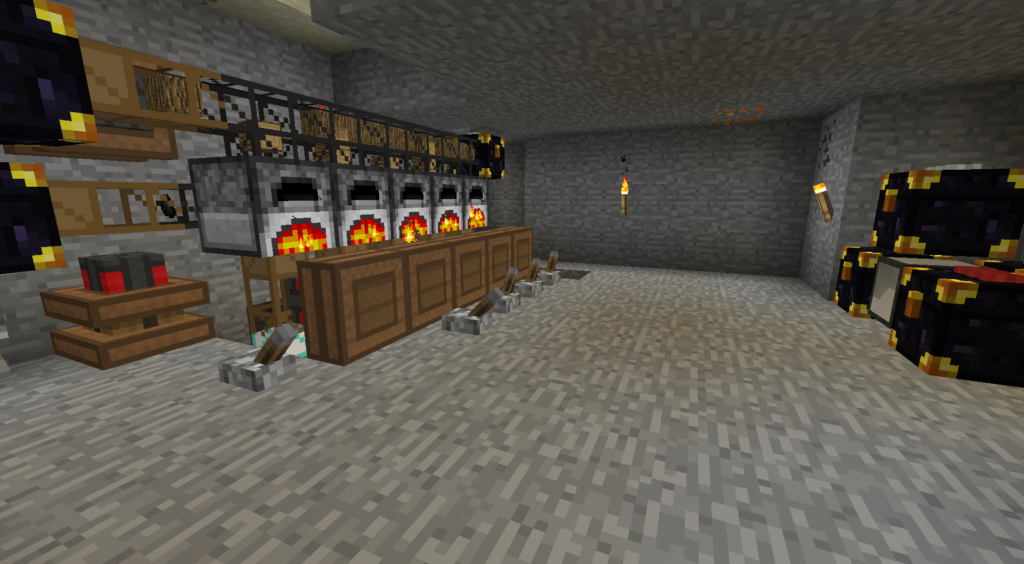
My second program was designed to take input from a chest, and craft an item from the input, then move all the excess into another chest. This was simple since the thing I needed to craft required one component item spread across the crafting grid (which is a surprisingly common case) this was easy to do by simply looping through an attempt to take items from the source until I had nine of them minimum, dividing it amongst the nine squares, and then crafting the item before sending everything into the output chest. If only things were that easy in real life!
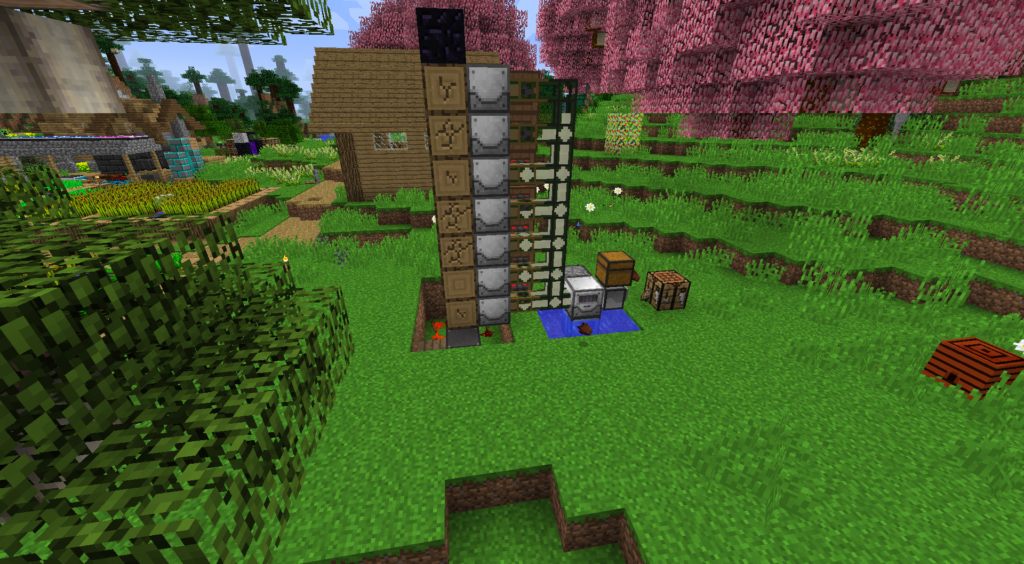
Join me next time when I take everything to a level far too complicated by making a NodeJS program interface with the turtles to automate my warehouse management for me, and another to manage mining dangerous long tunnels that feed into the warehouse system.
Program One
state = 0 -- 0 means the turtle is facing the intake chest
-- 1 is the clean output
-- 2 is fuel
function chance()
return math.floor(math.random()*2) ==1
end
function left()
state = state +1
turtle.turnLeft()
end
function right()
state = state - 1
turtle.turnRight()
end
function eat()
while state ~=0
do
if state > 0 then
right()
else
left()
end
end
while(turtle.getItemDetail(1) == nil)
do
turtle.suck()
end
distribute()
end
function distribute()
if turtle.getItemDetail(1).name == "minecraft:coal" then
good = chance()
if good then
left()
turtle.drop()
right()
else
left()
left()
turtle.drop()
right()
right()
end
else
if turtle.getItemDetail().name == "minecraft:log" then
turtle.dropUp()
else
left()
turtle.drop()
right()
end
end
eat()
end
eat()
Program 2
state = 0
function canCraft()
turtle.select(1)
return turtle.getItemCount() >= 9
end
function clearSlots()
for i=2,16,1
do
if turtle.getItemCount(i) ~= 0 then
turtle.select(i)
turtle.dropUp()
end
end
turtle.select(1)
end
function placeCrafting()
count = math.floor(turtle.getItemCount() /9)
print("There should be " .. count .. " rubber crafted")
turtle.transferTo(2,count)
turtle.transferTo(3,count)
turtle.transferTo(5,count)
turtle.transferTo(6,count)
turtle.transferTo(7,count)
turtle.transferTo(9,count)
turtle.transferTo(10,count)
turtle.transferTo(11,count)
end
function craft()
print("Crafting!")
clearSlots()
placeCrafting()
turtle.select(16)
turtle.craft()
turtle.dropUp()
turtle.select(1)
end
while (state == 0)
do
if canCraft() == false then
turtle.suck()
else
craft()
end
end