Today I coded some LED strip stuff.
Specifically: I’ve been working on a gift for my grandmother. It’s a multicolored LED lamp/trophy thing. It’s just supposed to be a cool gift nothing fancy.
The housing is 3D printed in PLA, and PETG for the clear parts since my Clear PLA was giving me lots of trouble. The code was developed in the course of a few hours, same with the design.
There are slight issues with the design. One of the major parts is that it’ll be 100% glued together since I didn’t engineer any snap fittings or anything fancy. Just glue and some prayers that it sticks together. Not my finest work but this was an afterthought project since I had already ordered something for my Grandmother but my own Mother insisted I make something for her instead.
The code itself runs off of a ESP32 development board. The board’s Micro USB header will be used for power and run a NEOPIXEL ARGB light strip for the lighting.
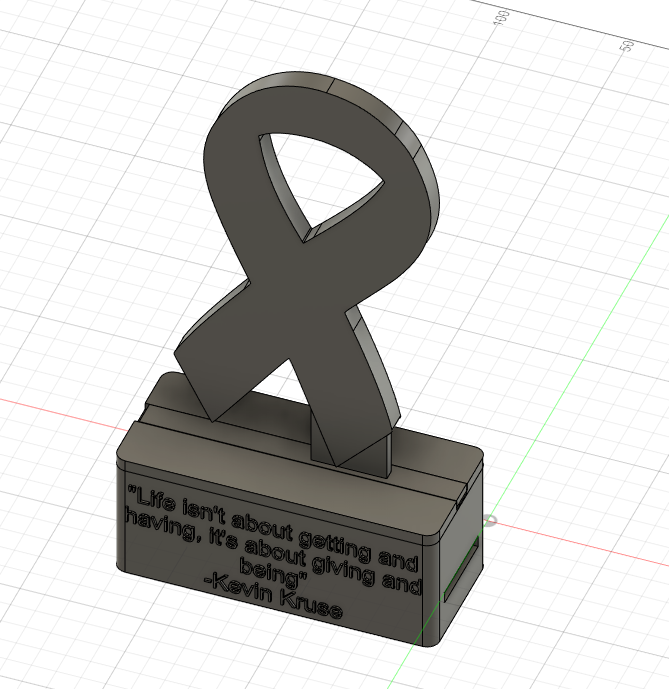
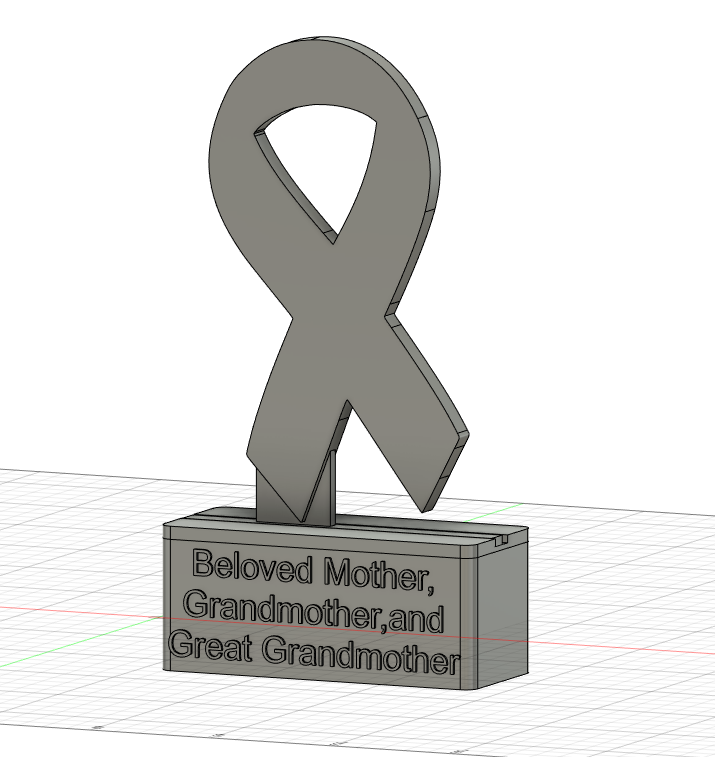
#include <Arduino.h>
#include <FastLED.h>
#define STRIP_SIZE 18 // Length of the LED Strip
#define FPS 60 // Frames per second, speed is tied to FPS
CRGB leds [STRIP_SIZE]; // Creating the LEDS array
int breatheCounter = 0;
bool breatheState = false;
int rainbowStart = 0;
bool twinkleBools[STRIP_SIZE];
int twinkleBright[STRIP_SIZE];
int myTime = 0;
int minsTillChange = 5;
int myTimeTillChange = (FPS*60*minsTillChange);
enum state
{
pink,
twinkle,
pinkle,
breathe,
rainbow,
dazzle
};
state loopstate = state::dazzle;
void setup() {
// put your setup code here, to run once:
FastLED.addLeds<NEOPIXEL, 22>(leds, STRIP_SIZE);
Serial.begin(115200);
twinkleBools[0] = true;
twinkleBools[1] = !true;
twinkleBools[2] = true;
twinkleBools[3] = !true;
twinkleBools[4] = !true;
twinkleBools[5] = true;
twinkleBools[6] = !true;
twinkleBools[7] = true;
twinkleBools[8] = !true;
twinkleBools[9] = true;
twinkleBools[10] = true;
twinkleBools[11] = !true;
twinkleBools[12] = true;
twinkleBools[13] = !true;
twinkleBools[14] = !true;
twinkleBools[15] = !true;
twinkleBools[16] = true;
twinkleBools[17] = true;
twinkleBright[0] = 20;
twinkleBright[1] = 40;
twinkleBright[2] = 50;
twinkleBright[3] = 30;
twinkleBright[4] = 90;
twinkleBright[5] = 200;
twinkleBright[6] = 150;
twinkleBright[7] = 170;
twinkleBright[8] = 60;
twinkleBright[9] = 70;
twinkleBright[10] = 10;
twinkleBright[11] = 0;
twinkleBright[12] = 250;
twinkleBright[13] = 220;
twinkleBright[14] = 120;
twinkleBright[15] = 110;
twinkleBright[16] = 80;
twinkleBright[17] = 20;
}
void twinkleF()
{
for (int i = 0; i<STRIP_SIZE;i++)
{
if (twinkleBools[i])
{
twinkleBright[i] +=10;
if (twinkleBright[i] >= 230)
{
twinkleBools[i] = false;
}
}
else
{
twinkleBright[i] -=10;
if (twinkleBright[i] <= 0)
{
twinkleBools[i] = true;
}
}
leds[i] = CRGB(twinkleBright[i],twinkleBright[i],twinkleBright[i]);
}
}
void pinkleF()
{
for (int i = 0; i<STRIP_SIZE;i++)
{
if (twinkleBools[i])
{
twinkleBright[i] +=10;
if (twinkleBright[i] >= 150)
{
twinkleBools[i] = false;
}
}
else
{
twinkleBright[i] -=10;
if (twinkleBright[i] <= 60)
{
twinkleBools[i] = true;
}
}
leds[i] = CRGB(twinkleBright[i],twinkleBright[i]/6,twinkleBright[i]/6);
}
}
void pinkF()
{
for (int i = 0; i<STRIP_SIZE;i++)
{
leds[i] = CRGB::DeepPink;
}
}
void breatheF()
{
if (breatheState)
{
breatheCounter++;
if (breatheCounter >= 230)
{
breatheState=false;
}
}
else
{
breatheCounter--;
if (breatheCounter <= 0)
{
breatheState=true;
}
}
CRGB col = CRGB(breatheCounter,breatheCounter,breatheCounter);
for (int i = 0; i<STRIP_SIZE;i++)
{
leds[i] = col;
}
}
int IntLerp(double one, double two, double percent)
{
return one + percent *(two - one);
}
CRGB rainbowColor(int myInt)
{
if (myInt > 0 && myInt<=100) // Red To Yellow
{
return CRGB(
IntLerp( 255, 255, (double) myInt/100),
IntLerp(0, 216, (double) myInt/100),
IntLerp(0, 0, (double) myInt/100)
);
}
else if (myInt > 100 && myInt<=200) // Yellow to Green
{
myInt -= 100;
return CRGB(
IntLerp( 255, 0, (double) myInt/100),
IntLerp(216, 255, (double) myInt/100),
IntLerp(0, 33, (double) myInt/100)
);
}
else if (myInt > 200 && myInt<=300) // Green to Blue
{
myInt -= 200;
return CRGB(
IntLerp( 0, 0, (double) myInt/100),
IntLerp(255, 38, (double) myInt/100),
IntLerp(33, 255, (double) myInt/100)
);
}
else if (myInt > 300 && myInt<=400) // Blue to Purple
{
myInt -= 300;
return CRGB(
IntLerp( 0, 78, (double) myInt/100),
IntLerp(38, 0, (double) myInt/100),
IntLerp(255, 255, (double) myInt/100)
);
}
else if (myInt > 400 && myInt<=500) // Purple to Red
{
myInt -= 400;
return CRGB(
IntLerp( 78, 255, (double) myInt/100),
IntLerp(0, 0, (double) myInt/100),
IntLerp(255, 0, (double) myInt/100)
);
}
return CRGB::Red;
}
void rainbowF()
{
rainbowStart++;
if (rainbowStart >= 499)
{
rainbowStart = 0;
}
CRGB col = rainbowColor(rainbowStart);
for (int i = 0; i<STRIP_SIZE;i++)
{
leds[i] = col;
}
}
void dazzleF()
{
if (rainbowStart >= 499)
{
rainbowStart = 0;
}
rainbowStart+=5;
int rain = rainbowStart;
for (int i = 0; i<STRIP_SIZE;i++)
{
if (rain >= 499)
{
rain = 0;
}
leds[i] = rainbowColor(rain);
rain+=10;
}
}
void loop() {
myTime++;
switch (loopstate)
{
case state::pink: pinkF(); break;
case state::twinkle: twinkleF(); break;
case state::pinkle: pinkleF(); break;
case state::breathe: breatheF(); break;
case state::rainbow: rainbowF(); break;
case state::dazzle: dazzleF(); break;
}
// put your main code here, to run repeatedly:
FastLED.show();
if (myTime<myTimeTillChange)
{
loopstate = state::pink;
} else if (myTime>= myTimeTillChange && myTime < myTimeTillChange*2)
{
loopstate = twinkle;
}else if (myTime>= myTimeTillChange*2 && myTime < myTimeTillChange*3)
{
loopstate = pinkle;
}else if (myTime>=myTimeTillChange*3 && myTime < myTimeTillChange*4)
{
loopstate = breathe;
}else if (myTime>=myTimeTillChange*4 && myTime < myTimeTillChange*5)
{
loopstate = rainbow;
}else if (myTime>=myTimeTillChange*5 && myTime < myTimeTillChange*6)
{
loopstate = dazzle;
}
else
{
loopstate = pink;
myTime = 0;
}
Serial.println(myTime);
delay(1000/FPS);
}